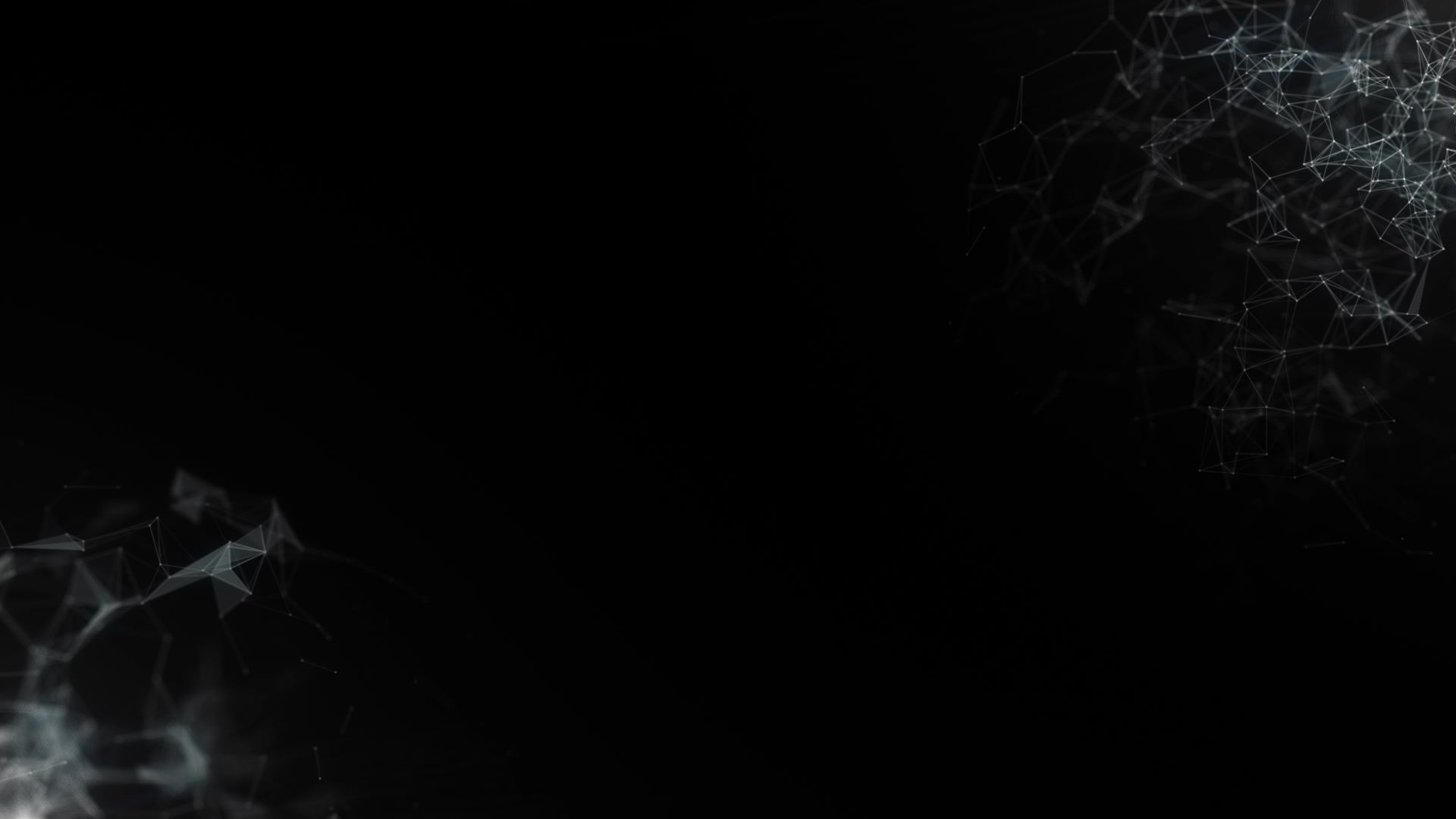
Programs For Bluej
PALINDROME NUMBER:
Program to check number entered is palindrome or not. A palindrome number is one that reads the same from left
and right eg. 1221, 323, 111, etc.
import java.io.*;
class palin
{
public static void ispalin(int num)
{
int s=0, quo=num, rem=0;
while ( quo>0)
{
rem=quo%10;
quo/=10;
s=s*10+rem; //reverse num
}
if(s==num)/check reverse and original number are same
{
System.out.println(num+ ” is palindrome”);
}
else
{
System.out.println(num+ ” is not palindrome”);
}
}//function ends
}//class ends
PERFECT NUMBER:
Program to find a number is perfect or not . A perfect number is one that is equal to the sum of the cubes of its digits eg. 6(1+2+3), 28(1 + 2 + 4 + 7 + 14), 128, 8128, etc.
Program should continue till the user wants.
import java.util.Scanner;
public class Perfect{
public void check() {
Scanner scan=new Scanner(System.in);
String ch=””;
int sum, n;
do {
System .out.println(“Enter an integer”);
n=scan.nextInt();
sum=0;
for(int i=1; i
if(n%i==0) //to check i is a factor of n or not
sum+=i; //find sum of factors
}//for ends
if(sum==n)
System.out.println(n+” is a perfect number”);
else
System.out.println(n + ” is not a perfect number”);
System.out.println(“Press y to continue, n to stop”);
ch=scan.next();
}while(ch.equalsIgnoreCase(“Y”));
}//method ends
} //class ends
ARMSTRONG NUMBER:
Program to check a number is armstrong or not. An armstrong number is one that is equal to the sum of the cube of its digits eg. 153 ( 1+ 125+27) , 370 (27+343), 371 (27+ 343+1) , etc.
import java.util.Scanner;
public class Armstrong {
void func() {
int n, tot=0,rem,quo;
Scanner scan=new Scanner(System.in);
n=scan.nextInt();
quo=n;
while(quo!=0) {
rem=quo%10;
tot=tot+rem * rem * rem;
quo=quo/10;
}// loop ends
if(tot==n)
System.out.println(“Armstrong”);
else
System.out .println(“Not Armstrong”);
}//method ends
} // class ends
Factorial of a given number:
Program to find factorial of a number entered by the user. Factorial is represented by the symbol ! eg. 5! = 5 * 4 * 3 * 2 * 1 = 120
import java.util.Scanner;
public class Factorial {
public void findfactorial() {
Scanner scan=new Scanner(System.in);
int f=1, i, n; // f to find factorial, i to generate natural nos. till n
n= scan.nextInt();
for ( i= 1; i<=n; i++)
f*=i;
System.out.println(” factorial of “+ n + ” is “+ f)
}// method ends
} // class ends
Factors of a Number:
Program to find factors of a given number eg factors of 12 are 1, 2, 3, 4, 6, 12
import java.util.Scanner;
class Factors{
void findfactors(){
Scanner scan = new Scanner(System.in);
int n=scan.nextInt();
System.out.println(“factors of “+ n+ ” are: “);
for(int i= 1; i<=n; i++) {
if(n% i==0)
System.out.println(i);
}}}
Prime Factors of a Number:
Program to find and print all prime factors of a number. eg prime factors of 12 are 2, 3 prime factors of 20 are 2, 5
public class PrimeFactors {
public void findPrimeFactors(int n) {
int c=0;
System.out.println(“Prime factors ” + n + ” are : ” );
for(int i=2; i<=n ; i++) {
if(n%i==0) {// check if i is a factor of n or not
c=0;
for(int j=2; j <i; j++) {// to check i is prime or not
if(i%j==0) {
c++;
break;
}//end if
}//end inner for
if(c==0) // if i is a prime factor
System.out.print(i + ” “);
} //end outer if
} //end outer for
}//end method
} //end class
Reverse of a Number:
Program to find and print reverse of a number eg reverse of 1367 = 7631
public class Reverse {
void func(int n) {
int rem,quo=n,rev=0;
while(quo!=0)
{
rem=quo%10;
rev=rev*10+rem;
quo=quo/10;
}
System.out.println(rev);
} }
Highest Common Factor(HCF/GCD):
Program to find the Greatest Common Divisor of two numbers entered by the user
public class HCF {
public void findHcf(int a, int b) {
int h=1, small=0;
if(a<b) //find smaller of two
small=a;
else
small=b;
for(int i=1;i<=small; i++) {
if(a%i==0 && b%i==0)
h=i;
}
System.out.println(“HCF of “+ a+ “and “+ b+ ” = ” + h);
} }
Lowest Common Divisor (LCM) :
Program to find the LCM of two numbers entered by the user
public class LCM {
public void findLCM(int a, int b)
{
int l=1;
for(int i=1; i<=(a*b); i++) {
if(i%a==0 && i%b==0)
{
l=i;
break;
}//if ends
} //for ends
System.out.println(“LCM of ” + a+ ” and “+ b + ” = ” + l);
}//method ends
}//class ends
Table of a number(First 10 multiples):
Program to print the first 10 multiples of the number entered by the user
public class Table {
public void printTable(int n) {
for( int i=1; i<=10; i++) {
System.out.println(n+ ” * “+ i + ” = “+ n*i);
} } }
Binary number to its Decimal equivalent:
Program to convert a binary number entered by the user to its decimal equivalent
public class BinaryToDecimal {
void func(int n)
int rem=0, quo=n, po=0, bin=0;
double p=0;
while(quo!=0) {
rem=quo%10;
p=Math.pow(2,po);
bin =bin+rem*(int) p;
po++;
quo=quo/10;
}
System.out.println(bin);
}}
Decimal number to its Binary equivalent:
Program to convert a decimal number entered by the user to its binary equivalent
//program to convert a decimal number to its binary equivalent
import java.util.Scanner;
public class DecimalToBinary
{
void findBinary()
{
//Declaring variables
Scanner scan = new Scanner(System.in);
int deci, rem=0, quo, i=0, bin=0;
//Taking inputs
System.out.println(“Enter a decimal number”);
deci= scan.nextInt();
//finding binary equivalent
quo=deci;
while(quo!=0)
{
rem=quo%2;
bin+= rem*(int)Math.pow(10,i);
quo=quo/2;
i++;
}//end while
//Displaying output
System.out .println(“Binary equivalent of Decimal number “+deci+” = “+bin);
}//endmethod
}//endclass
Roots of Quadratic Equation:
// to find roots of a quadratic equation
public class Roots
{
public void findRoots(int a, int b, int c)
{
double x1, x2;
if(a!=0)
{
int d = b*b- 4*a*c;
if(d>=0)
{
x1=(-b+Math.sqrt(d)/(2*a));
x2=(-b-Math.sqrt(d)/(2*a));
System.out.println(” Two roots are ” + x1 + ” and ” + x2);
if(d>0)
System.out.println(” Roots are real and unequal “);
else
System.out.println(” Roots are real and equal “);
}//inner if ends
else
System.out.println(” Roots are imaginary “);
}//outer if ends
else
System.out.println(” Not a quadratic equation “);
}//method ends
} // class ends
Print Factors of n and Sum of its Even and Odd Factors
// to print factors of n and find sum of its even and odd factors
public class SumEvenOddfactors
{
public void findSum(int n)
{
int even=0, odd=0;
System.out.println(“Factors of “+n + ” are\n”);
for(int i=1; i<=n; i++)
{
if(n%i==0)
{
System.out.print(i+” “);
if(i%2==0)
even+=i;
else
odd+=i;
}
}
System.out.println(“\nSum of even factors = ” + even);
System.out.println(“Sum of odd factors = ” + odd);
}
}
Print Factors of n and their Count
// to print factors of n and their count
public class CountFactors
{
public void count(int n)
{
int c=0;
System.out.println(“Factors of “+n+” are “);
for(int i=1; i<=n; i++)
{
if(n% i==0)
{
System.out.print(i+” “);
c++;
}
}
System.out.println(“\nNo. of factors = ” + c);
}
}
Print Factors of N and their Sum
// to print factors of n and their sum
public class SumFact
{
public void findSum(int n)
{
int sum=0;
System.out.println(“factors of “+n +” are “);
for(int i=1;i<=n;i++)
{
if(n%i==0)
{
System.out.print(i+” “);
sum+=i;
}
System.out.println(“\nSum of factors of ” +n+ ” is ” + sum);
}
}
Print All Prime factors of a given Number
//To find all prime factors of a given number
public class ALLPrimeFactors
{
void findPrimeFactors(int n)
{
int i,j,c=0;
for(i=1;i<=n;i++)
{
if(n%i==0)
{
c=0;
for(j=1;j<=i;j++)
{
if(i%j==0)
c++;
}
if(c==2)
System.out.println(i);
}
}
}
}
Print Sum of Even and Odd Digits of a given Number
//to find sum of even and odd digits of a number
public class EvenOdd
{
void func(int n)
{
int odd=0, even=0,rem,quo=n;
while(quo!=0)
{
rem=quo%10;
if(rem%2==0)
even=even+rem;
else
odd=odd+rem;
quo=quo/10;
}
System.out.println(“Sum of even digits= “+ even);
System.out.println(“Sum of odd digits= “+ odd);
}
}
Print Sum of First and Last Digits Of a Number
//to find sum of first and last digits of a number
public class SumFirstLast
{
void func(int n)
{
int tot=0,rem=0,quo=n, first=0;
first=quo%10;
while(quo!=0)
{
rem=quo%10;
quo=quo/10;
}
tot=first+rem;
System.out.println(tot);
}
}
Print Sum of Prime Digits of a Number
//To find sum of prime digits of a numbers
public class SumPrimeDigits
{
void sumPrimeDigits(int n)
{
int rem=0,j,c=0, tot=0;
while (n!=0)
{
rem=n%10;
n=n/10;
c=0;
for(j=1;j<=rem;j++)
{
if(rem%j==0)
c++;
}
if(c==2)
tot+=rem;
}
System.out.println(tot);
}
}
Print Product of Digits of a Number
//to find product of digits of a number
public class ProductOfDigits
{
void func(int n)
{
int pro=1,rem=0,quo=n;
while(quo!=0)
{
rem=rem%10;
pro=pro*rem;
quo=quo/10;
}
}
}
Print Sum of Digits of a Number
//to find sum of digits of a number
public class SumOfDigits
{
void func(int n)
{
int tot=0,rem,quo=n;
while(quo!=0)
{
rem=quo%10;
tot=tot+rem;
quo=quo/10;
}
System.out.println(tot);
}
}
Print Sum of Digits of a Number till it becomes a single digit number
//to find sum of digits of a number till it becomes a single digit number
public class Sum
{
void func(int n)
{
int tot=0,rem,quo=n;
while(n>9)
{
tot=0;
while(quo!=0)
{
rem=quo%10;
tot=tot+rem;
quo=quo/10;
}
n=tot;
System.out.println(tot);
}
}
}
SPECIAL NUMBERS:
A Special number is one where the sum of the factorial of each digit of the number is equal to the number itself. This code checks whether the number entered by the user is special or not.
import java.io.*;
public class SpecialNumber
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
int n,sum=0;
int i,j;
public void checkSpecial() throws Exception
{
System.out.println(“Enter the number:”);
n=Integer.parseInt(br.readLine().trim());
for(i=n;i>0;i=i/10)
{
sum=sum+fact(i%10);
}
if(sum==n)
System.out.println(n+ “is a special number.”);
else
System.out.println(n+ “is not a special number.”);
}
//to find factorial
int fact(int x)
{
int f=1;
for(j=2;j<=x;j++)
f=f*j;
return f;
}}
EXPLANATION:
Each digit of the entered number is extracted using a for loop and the digit is passed to a function ‘int fact (int)’ where factorial of the digit is calculated and returned. The addition value of the factorial values are stored in a variable and at the end the number entered and the sum of factorial values are checked for equality.
……………………………………………………………………………………………………………………………………………………………………….
Program to print the nth prime number
class PrimeNumber
{
void findPrime(int n)
{
int c, i=1, count=0;
System .out. println( n+”th prime numbers are:”);
while (count < n)
{
c=0;
for(int x=1; x<=i; x++)
{
if(i%x==0)
c++;
}
if (c==2)
{
System .out.print(i +” “);
count++;
}
i++;
}//end for
}//end method
}//end class
Print star pattern in java
class StarTriangle { public static void main(String[] args) { int i,j,k; for(i=1; i<=5; i++) { for(j=4; j>=i; j--) { System.out.print(" "); } for(k=1; k<=(2*i-1); k++) { System.out.print("*"); } System.out.println(""); } } }
Syntax to compile and run java program
Syntax
for compile -> c:/>javac StarTriangle.java for run -> c:/>java StarTriangle
Explanation of Code
-
System.out.print("....."): are used for display message on screen or console but cursor not move in new line.
-
System.out.println("....."): are used for display message on screen or console cursor move in new line.
Example
class Star { public static void main(String[] args) { int i, j, k; for(i=5;i>=1;i--) { for(j=5;j>i;j--) { System.out.print(" "); } for(k=1;k<(i*2);k++) { System.out.print("*"); } System.out.println(); } }
Example
class Star { public static void main(String[] args) { int i,j; for(i=1; i<=6; i++) { for(j=1; j<i; j++) { System.out.print("*"); } System.out.println(); } } }
Example
class Star { public static void main(String[] args) { int i, j; for(i=5;i>=1;i--) { for(j=1;j<=i;j++) { System.out.print("*"); } System.out.println(); } } }
Example
class Star { public static void main(String[] args) { int i, j, k; for(i=5;igt;=1;i--) { for(j=1;jlt;i;j++) { System.out.print(" "); } for(k=5;k>=i;k--) { System.out.print("*"); } System.out.println(); } } }
Example
class Star { public static void main(String[] args) { int i, j, k; for(i=5;i>=1;i--) { for(j=5;j>i;j--) { System.out.print(" "); } for(k=1;k<=i;k++) { System.out.print("*"); } System.out.println(); } } }
Example
class Star { public static void main(String[] args) { int i, j, k; for(i=1;i<=5;i++) { for(j=i;j<5;j++) { System.out.print(" "); } for(k=1;k<(i*2);k++) { System.out.print("*"); } System.out.println(); } for(i=4;i>=1;i--) { for(j=5;j>i;j--) { System.out.print(" "); } for(k=1;k<(i*2);k++) { System.out.print("*"); } System.out.println(); } } }